Installing Widget
Doris Widget is a JavaScript SDK that enables simple and efficient integration of our widget into your online shop.
Requirements
- An API Key
- Products imported and approved by the Doris team
Installation and initialization
To include the Doris Widget SDK directly in your HTML, use the following method:
1<script
2 defer
3>
4 (function () {
5 const API_KEY = 'YOUR API KEY';
6
7 function handleBuyButton(skus) {
8 yourPlatformAddToCartFunction(...skus)
9 }
10
11
12 function handleScriptLoaded() {
13 injectDorisButton();
14 }
15
16 function injectDorisSDK() {
17 const script = document.createElement('script');
18 script.type = 'text/javascript';
19 script.async = true;
20 script.onload = handleScriptLoaded;
21 script.src = 'https://mix.doris.mobi/doris-widget.js';
22 document.head.appendChild(script);
23
24 const dorisWidget = document.createElement('div');
25 dorisWidget.id = 'doris-widget';
26 document.body.appendChild(dorisWidget);
27 }
28
29 function initializeDorisWidget() {
30 window.DorisWidget.init({
31 apiKey: API_KEY,
32 theme: { colors: { primary: '#000000' } },
33 position: { x: 'left' },
34 splashImage: 'https://example.com/your-splash-image.jpg',
35 language: 'en',
36 handleBuyButton,
37 });
38 }
39
40 injectDorisSDK();
41 })();
42</script>
SDK API Methods
Before executing any of the following methods, ensure that the widget's SDK has been fully downloaded. In the example above, we added a callback that triggers the
init
method during the onload
event of the SDK script configuration.DorisWidget.init(options)
Parameters:
options
(Object): A configuration object with the following properties:apiKey
(String, required): The API key provided.theme
(Object, optional): A theme configuration object. Currently, you can configure only the primary color with the following properties:{ color: primary: '#000000' }
position
(Object, optional): An object for configuring the horizontal position of the widget on the page:{ x: ‘left' }
the x property could beleft
orright,
default value isright.
splashImage
(String, optional): URL of the image displayed in the SplashScreen. It must be served over HTTPS. If not provided, the Widget will use a default Doris image. Click here to download the .psd file template for constructing the splash image.language
(String, optional): Should be one ofen
,pt
, ores
. If not provided, the Widget will use the language attribute from the HTML document. If the document language is not supported, the default language will be English.
DorisWidget.injectButton(options)
The
injectButton
function is responsible for inserting a button that opens the widget.Parameters:
options (Object):
A configuration object with the following properties:apiKey
(String, required): The API key provided.selector
(String, required): A selector pointing to the container (div, section, etc.) where the button will be injected.skus
(Array of Strings, optional): A list of product SKUs. If the values are valid, the Widget will perform a try-on with the products when opened.validateSku
(Boolean, optional): Determines whether the button should appear only if the products specified in theskus
key are available in Doris. If set tofalse
, the button will render without validation. We recommend setting this totrue
for product detail pages andfalse
for landing pages or banners.backgroundImages
(String, optional): An array of URLs of images to be displayed within the injected button. These images are provided by Doris, but if you wish to use a customized image from your catalog, please contact Doris Support.description
(String, optional): A string to be displayed next to the button. If not provided, default text will be shown.showBadge
(Boolean, optional): Determines whether a 'NEW' tag will be displayed in red at the top left corner of the button. Doris recommends enabling the 'NEW' tag for increased visibility of the widget.useNewTrigger
(Boolean, optional): Determines the layout of the button. Enable this option If you want to use DORIS layout,. If you prefer to use your own layout, do not enable this option. Customization is not allowed in DORIS layout.
Implementation example:
1// this needs a element with 'doris-trigger-wrapper' id in the page.
2// like this: <div id="doris-trigger-wrapper"></div>
3
4window.DorisWidget.injectButton({
5 apiKey: 'YOUR API KEY',
6 selector: '#doris-trigger-wrapper',
7 skus: ['101010'],
8 validateSku: true,
9 backgroundImages: [
10 'https://doris-media-production.s3.sa-east-1.amazonaws.com/mix-static-assets/doris-trigger-default-images/F09.png',
11 'https://doris-media-production.s3.sa-east-1.amazonaws.com/mix-static-assets/doris-trigger-default-images/F13.png',
12 'https://doris-media-production.s3.sa-east-1.amazonaws.com/mix-static-assets/doris-trigger-default-images/M01.png',
13 'https://doris-media-production.s3.sa-east-1.amazonaws.com/mix-static-assets/doris-trigger-default-images/M02.png',
14 ],
15 description: 'This is a custom description',
16 showBadge: true,
17})
18
The
order
method is responsible for generating a tracking tag for the products that have been purchased. It should be executed by the partner after payment confirmation for an order, passing a list of SKUs for all the products purchased by the user.This step is crucial and mandatory for the approval of your installation. The data collected through this tracking will be used as outlined in our contract, privacy policy, and terms of use.Parameters:
options
(Object): A configuration object with the following properties:id
(Number, required): The order numberproducts
(Array of Objects, required): An array of product objects, each containing:sku
(String, required): The product SKUidentifier
(String, optional): The product identifierquantity
(Number, required): The quantity of ordered productstotal
(Number, required): The total value of the items (unit price * quantity)
value
(Number, required): The total value of the ordercurrency
(String, required): The currency used (in standard ISO 4217 format). The default value is BRL (Brazilian Real).
Implementation example:
1window.DorisWidget.order({
2 id: 1243,
3 value: 389.9,
4 currency: "BRL",
5 products: [
6 {
7 sku: "101011",
8 quantity: 2,
9 total: 779.8,
10 identifier: "101010",
11 },
12 ],
13});
14
DorisWidget.start(options)
This method opens the widget for a try-on experience. We recommend using it for clicks on banners in advertising campaigns, for example.
Parameters:
options
(Object): A configuration object with the following properties:skus
(Array of Strings, optional): A list of SKUs that will be dressed on the model, if they exist.defaultGender
(String, optional): Valid values aremale
orfemale
. This specifies the gender for the Widget's initial display if no SKU is identified for dressing. If not provided, the default gender chosen by the partner will be used.
Implementation example:
1window.DorisWidget.start({
2 skus: ['101010'],
3 defaultGender: 'male'
4});
5
DorisWidget.verify(options)
The
verify
function checks if the specified product SKU exists within Doris. It must be executed on all pages where the Widget can be initiated by the user. This function returns a boolean value (true
or false
).Parameters:
options
(Object): A configuration object with the following properties:apiKey
(String, required): The API key provided.sku
(String, required): The SKU of the product to be verified. If not provided, the function will always returntrue
.
Implementation example:
1const productIsValid = await window.DorisWidget.verify({
2 apiKey: 'YOUR API KEY',
3 sku: '101010',
4})
5
6
7console.log(productIsValid) // true or false
Installation process and key considerations
To include the Doris Widget SDK directly in your HTML, use the following method:
- Partners must implement a button in their application to trigger the Doris Widget within a webvie
- The widget will be triggered via a coded URL with URL parameters, allowing the Doris Widget to open automatically. For example: https://www.partner.com.br/dorispage/?dwoa=1&?dwskus=sku1&?dwview=1&?dwappuser=appUserId
- The webview should contain as few resources as possible to prevent unnecessary browsing by the consumer.
- Before displaying the webview, the application must request permission to access the camera, photo gallery, and audio, as these resources are utilized by the Doris Widget.
- It is essential for the Doris Widget to receive a user identifier from the app via the
dwappuser
URL parameter, as this identifier is used to track conversion tags. - Add the
allowsInlineMediaPlayback
property to the webview component to enable HTML5 videos to render inline (within the webview). - Enable caching and refrain from clearing local storage, as all Doris Widget data is stored there.
Example code
1<WebView
2 useWebKit
3 originWhitelist={['*']}
4 source={{uri: 'https://mix.doris.mobi?dwoa=1&dwview=1'}}
5 contentInsetAdjustmentBehavior={'always'}
6 allowsInlineMediaPlayback
7/>
- Partners must create a web page for the Doris Widget to open
- This page will serve as the foundation for opening and instantiating the Doris Widget in all webview calls originating from the app. It is crucial that the page is clean while also providing some user feedback information, similar to the example above.
- The page must have the Doris Widget SDK and initialization methods (INIT) already installed.
- Changes to the DorisWidget
- Upon receiving the query string from
dwview
, the Doris Widget will enter a specific mode that includes the following adjustments:- The DorisWidget will open in “full screen,” meaning it will not have rounded edges or borders.;
- The DorisWidget will hide the close button (“X” in the top right corner) to prevent user confusion.
- Upon receiving the query string from
- Partners must send conversion tags via the SDK specific to their application
- Doris will provide an SDK in the programming language of the partner's app to facilitate this. The conversion tag will include the same information as the
ORDER
method (detailed in our installation documentation) along with the app user identifier received via the query string in the Doris Widget call.- a) We currently have an SDK available for React Native web applications.
- Doris will provide an SDK in the programming language of the partner's app to facilitate this. The conversion tag will include the same information as the
- Partners must ensure they can receive and direct the corresponding deep link
- When a user browses on a desktop browser and uses the Doris Widget to take a photo, the Doris Widget will direct the user via QR code back to the product page they were on, automatically starting the Doris Widget. To achieve this, Doris Widget uses string queries similar to those provided earlier.
- According to Doris, the ideal user flow in the app should be as follows:
a) Open the app;
b) Be directed to the corresponding product page;
c) Have the Doris Widget open with the same string queries necessary for the webview, as described in the #1 item.
- Inhibiting the bag feedback Widget
- Objective: Prevent the widget from providing feedback.
Implementation steps:
a) Suggestion: After adding products to the cart, close the webview and provide appropriate feedback to the user.
- Objective: Prevent the widget from providing feedback.
- Modify the function passed to
handleByButton
(injected in the INIT method) to always returnfalse
.
Installing in a react-native app
The purpose of this document is to detail the installation process of the DorisWidget within a React Native e-commerce application.
The installation document is available at Installation and initialization.
Doris Widget is a browser-based solution, allowing it to be integrated into e-commerce applications via webview method.
Doris Widget is a browser-based solution, allowing it to be integrated into e-commerce applications via webview method.
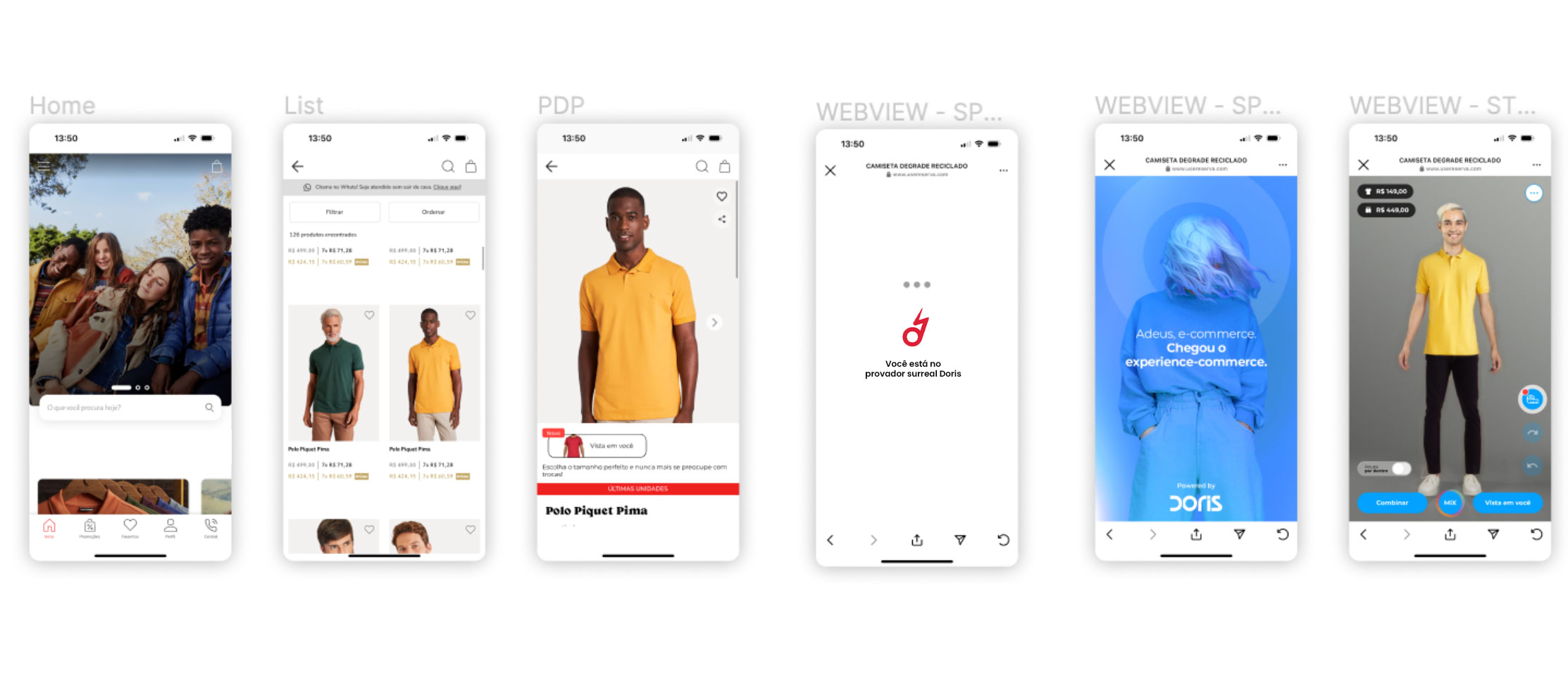